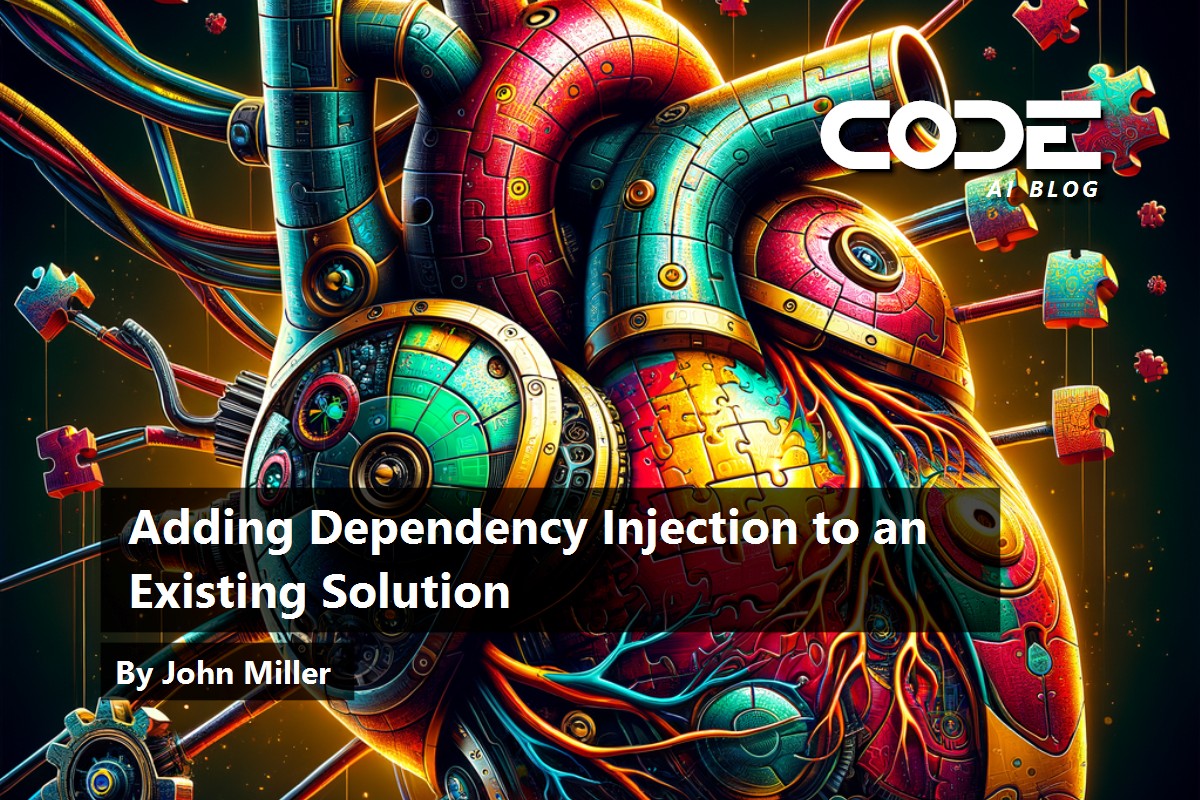
Adding Dependency Injection to an Existing Solution
by
| January 30, 2025John Miller explores the challenges and benefits of refactoring legacy code by integrating dependency injection (DI) to improve software maintainability and testability. He addresses common issues in legacy systems, such as tight coupling and lack of interfaces, which hinder the ability to conduct effective unit testing. John emphasizes the importance of DI and interfaces in creating flexible, modular, and isolated code components, thereby facilitating easier testing and maintenance. Using a practical example, he illustrates how DI can decouple service classes from specific implementations and discusses the role of tools like GitHub Copilot in automating and accelerating the refactoring process. By implementing DI, developers can enhance code flexibility, allowing for seamless integration of alternative components and improving overall software quality.
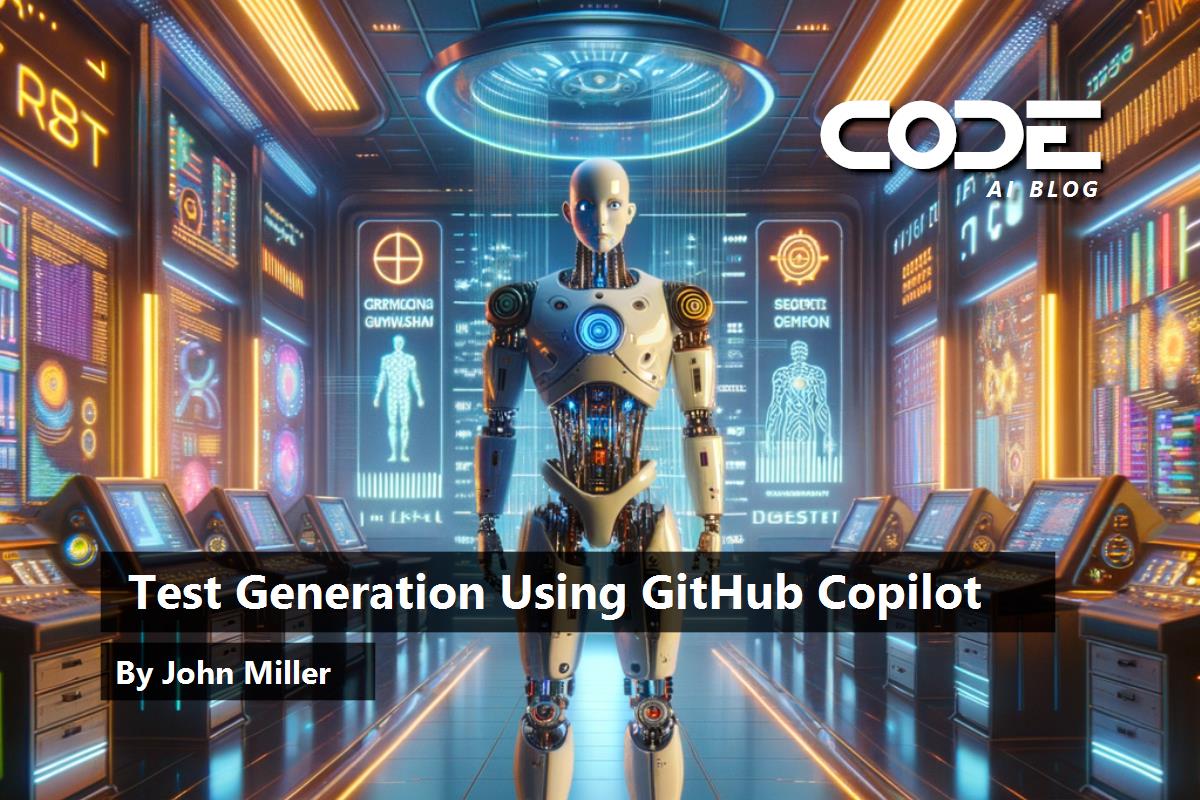
Test Generation Using GitHub Copilot
by
| December 13, 2024John's blog post "Test Generation Using GitHub Copilot," explores the strategic advantages of employing AI, specifically GitHub Copilot, for generating test automation in software development. Acknowledging the risks of integrating AI-generated code in production environments, John suggests that test automation provides a safer entry point due to its non-production deployment. Highlighting test automation's role in exposing design flaws and enabling refactoring, the post demonstrates how Copilot can effectively generate unit tests, using a complex C# BankAccount class as a case study. John goes on to show examples of the process of generating tests using Copilot’s integration with various IDEs, emphasizing that despite occasional test adjustment requirements, AI-enhanced test automation facilitates higher code coverage, efficiency, and reliability. John advocates for embracing AI tools like Copilot to enhance the development workflow, paving the way for more robust and maintainable software systems.
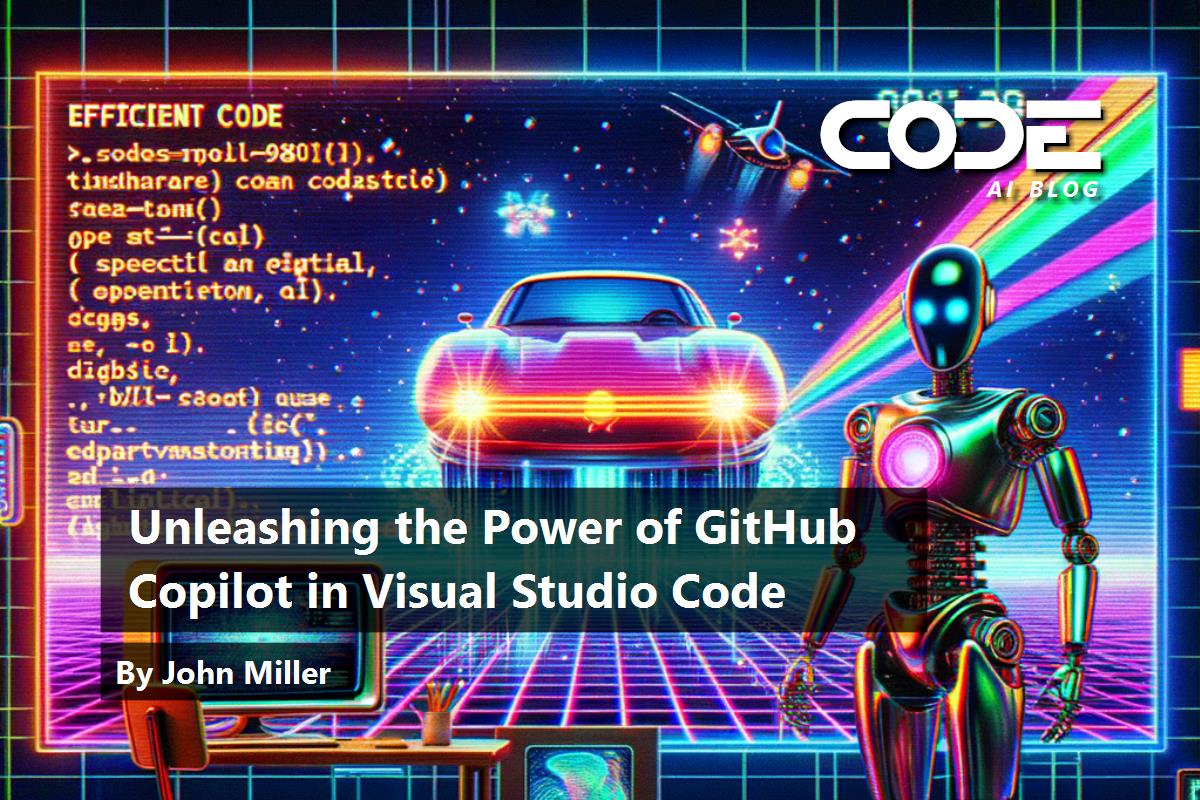
Unleashing the Power of GitHub Copilot in Visual Studio Code
by
| November 11, 2024John Miller explores the transformative potential of GitHub Copilot, an AI-powered coding assistant created by GitHub and OpenAI. Leveraging machine learning on vast amounts of public code, Copilot offers context-aware code suggestions, enhancing productivity for developers by streamlining tasks such as writing functions or debugging. John provides a guide to integrating GitHub Copilot with Visual Studio Code, covering installation, authentication, and features like autocomplete, context-aware coding, multiline completions, and example-based learning. He delves into the functionality of the @workspace command, Copilot's chat commands, and various integration features within VS Code. The post emphasizes Copilot's ability to optimize the coding process, allowing developers to focus on creative problem-solving by reducing the burden of writing boilerplate code and improving overall workflow efficiency.
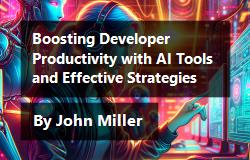
Boosting Developer Productivity with AI Tools and Effective Strategies
| 10/14/2024
John Miller explores how AI is transforming the tech industry by enhancing developers' capabilities rather than threatening their jobs. He emphasizes that developers who embrace AI can automate repetitive tasks, enhance coding efficiency, and focus on more strategic work. Key insights from McKinsey and Microsoft highlight AI's role in accelerating code documentation, optimization, and writing, while also promoting deep work by minimizing interruptions. Improved developer experiences lead to higher product quality and employee satisfaction. John advocates for adopting an AI mindset to maintain a competitive edge and plans to delve deeper into AI's impact on the software development life cycle in future posts.
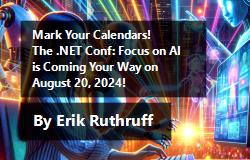
Mark Your Calendars! The .NET Conf: Focus on AI is Coming Your Way on August 20, 2024!
| 8/13/2024
In an upcoming virtual community event, .NET Conf: Focus on AI, set for August 20, 2024, CODE Magazine is excited to support readers as they learn about the integration of artificial intelligence tools and libraries within the .NET platform. This free online event aims to provide developers with a comprehensive introduction to leveraging AI in .NET, featuring insights from renowned speakers like Scott Hanselman and Stephen Toub. As a proud partner, CODE Magazine will offer digital subscriptions as part of a giveaway to enhance the attendee experience. Join us for a deep dive into AI and .NET, and potentially win a valuable subscription to CODE Magazine.
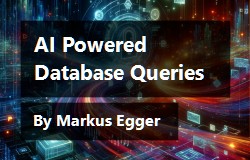
AI Powered Database Queries
| 7/16/2024
In his blog post "AI Powered Database Queries," Markus Egger introduces a groundbreaking feature that allows AI-driven natural language prompts to generate and execute complex SQL database queries. This innovation differs from traditional AI Copilot features, which typically handle smaller datasets in a conversational manner. Instead, Egger's approach leverages large language models (LLMs) such as GPT-4 to interpret user prompts, generate accurate SQL queries, and handle vast amounts of data. The implementation includes multiple layers of security checks and verification processes to ensure the generated queries are safe and meet the intended criteria. Egger argues that while some database vendors are working on integrating similar features, the custom, scenario-specific AI data dictionaries and rigorous security protocols his team employs offer a more secure and tailored solution. This advancement stands to significantly enhance how organizations can interact with and extract value from their large datasets.
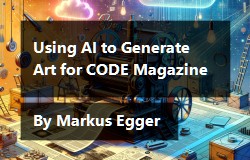
Using AI to Generate Art for CODE Magazine
| 6/19/2024
In his blog post, Markus Egger outlines the innovative use of AI to generate art for CODE Magazine, addressing the challenge of creating cost-effective, high-quality artwork for numerous articles and social media platforms. Markus describes a streamlined process involving multiple AI systems: an AI that generates article synopses, another that crafts creative and contextually relevant prompts, and an image generation AI (e.g., OpenAI's Dall-E 3) that produces original visuals. Further refinement and formatting are handled by an AI-driven tool called "Olympus Image Composer." Markus emphasizes that this technology not only optimizes resources but also expands artistic opportunities, countering concerns about job elimination by highlighting an overall increase in art-related employment. This post provides valuable insights for managers, decision-makers, and developers interested in leveraging AI for content creation and operational efficiency.
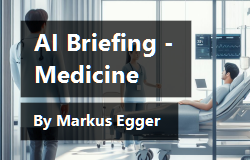
AI Briefing - Medicine
| 11/27/2023
This blog post highlights a recent presentation at a major U.S. medical institution, focusing on the integration of Artificial Intelligence (AI) in the medical industry. The post introduces the concept of "Executive Briefings on AI," tailored to the medical sector, encompassing hospitals and research applications. It delves into a scenario where AI assists doctors in emergency situations, even with limited prior knowledge of the patient's medical history. The presentation demonstrates a real-world example of AI in diagnosing medical emergencies, analyzing vital signs and medical history. A unique feature of this AI system is its multilingual capabilities, exemplified by diagnoses provided in different languages. The post emphasizes that such AI applications are not futuristic concepts but present-day realities. It aims to enlighten decision-makers in medical settings about the current potential and challenges of AI in healthcare.
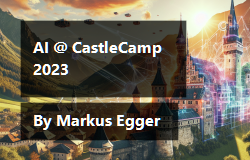
AI @ CastleCamp 2023
| 10/1/2023
CastleCamp 2023, a unique "unconference" in the Austrian Alps, united tourism professionals in the mesmerizing setting of Burg Kaprun Castle. Veering from the typical conference structure, attendees presented and voted on topics, fostering an environment of exchange and dialogue. Surprisingly, the dominating interest was the integration of Artificial Intelligence (AI) in the tourism sector. From employing technologies like ChatGPT and DALL-E for content generation to conceptualizing an AI-driven concierge for hotels, the event underscored the vast potential of AI applications in hospitality. Furthermore, the author's insights into integrating AI into business applications were met with significant interest, reaffirming the pervasive influence of AI across industries. The picturesque castle backdrop, juxtaposed with discussions on cutting-edge technology, made for an unforgettable experience.
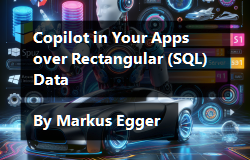
Copilot in Your Apps over Rectangular (SQL) Data
| 6/21/2023
In a recent State of .NET event, a remarkable demonstration showcased the capabilities of a custom Copilot utilizing Azure OpenAI. This large language model (LLM)-powered tool showcased its ability to navigate and reason over data across numerous SQL Server tables, challenging the perceived limitations of LLMs. By seamlessly integrating with a custom application, this Copilot unveiled the potential of intelligent machines and software working together to make informed decisions and optimize processes with unprecedented precision. This demo not only provided a glimpse into the future of technology but also emphasized the boundless possibilities of .NET and AI integration. The era of intelligent entities capable of learning, reasoning, and interacting with data has arrived, and the future promises even more exciting advancements.
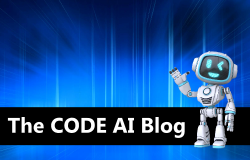
Welcome to the CODE Magazine Artificial Intelligence Blog!
| 6/7/2023
Welcome to the CODE Magazine Artificial Intelligence Blog, your go-to resource for everything AI! Led by our luminary founder, Markus Egger, we aim to demystify the complex world of AI for our diverse audience, from software developers to IT decision-makers. With a blend of engaging articles, practical applications, and a dash of humor, our blog covers a wide spectrum of AI topics. From basic concepts to cutting-edge research, we aim to make AI as accessible as your favorite programming language. So, buckle up for a thrilling ride through the transformative and exhilarating world of AI, right here at the CODE Magazine AI Blog!