Finding what you need in the Microsoft® Visual Studio® .NET documentation, which has over 45,000 topics, can be a daunting task. The Doc Detective is here to help, utilizing his investigative skills to probe the depths of the documentation.
Can't find what you're looking for? Just ask - if it's in there, I'll find it for you; if it isn't, I'll let you know that as well (and tell you where else you might go to find it).
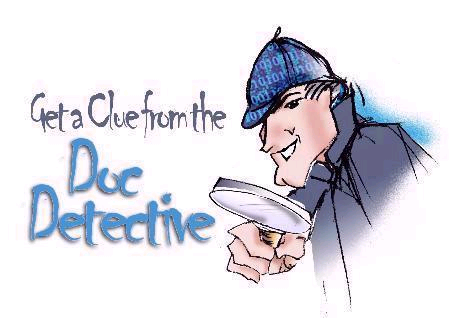
Have a question for the Doc? Send your questions for future columns to me at docdetec@microsoft.com.
Dear Doc Detective,
I should know how to do this, but I can't remember how to clear the entries from all of the text boxes on an entry form and then set the focus back to the first text box. Is there an example of the code to do this?
- Stuck in Stuckeyville
Dear Stuck,
Clearly this is a common task; however the Doc can't find anything in the docs that shows how to do this. I assume you are talking about clearing the contents of a group of TextBox controls. The following code should do the trick:
Dim i As Integer
For i = 0 To Me.Controls.Count - 1
Me.Controls(i).Text = 0
Next
Me.TextBox1.Focus()
Note that this will clear the text of all controls that have a Text property, including Labels. To avoid this, you can use the TypeOf method to check for TextBox controls:
Dim i As Integer
For i = 0 To Me.Controls.Count - 1
If TypeOf (Me.Controls(i)) Is TextBox Then
Me.Controls(i).Text = 0
End If
Next
Me.TextBox1.Focus()
Hope this clears things up!
- Doctor D
Dear Doc Detective,
I have an application created in Visual Basic which looks fine on my computer, but when I run it on a computer with a different screen resolution the size of my form and controls change. For example, the width of my form is 900 pixels on a screen with a resolution of 12 TwipsPerPixelX and 12 TwipsPerPixelY, but on a screen with a 15 TwipsPerPixelX screen resolution it is less than 850 pixels wide.
What's going on here? Am I crazy, or is this a bug?
-- Ruminating in Romania
Dear Ruminating,
I can't tell from the letter whether or not you are crazy, but it's not a bug. In Visual Basic .NET the standard unit of measurement is pixels; the TwipsPerPixel functions are no longer necessary. If you set the AutoScale property for a form to True (the default), the form should automatically display properly at any screen resolution.
There is a column in the MSDN Library that explains the ins and outs of scaling Windows Forms?although it was written for Visual C#, all of the same principles apply. Just look for "Windows Forms Layout," and before you know it your forms will be appearing just fine.
- the Doc
Dear Doc Detective,
I need to write code in Visual Basic .NET to populate a TreeView with the items selected in a folder browser dialog box, and display all of the subdirectories and files when I choose the main folder.
I'm also trying to write code to copy a selected node from the TreeView to a second TreeView when the user clicks a button. Is there anything that describes how to do this?
-- Out on a Limb in Lincoln
Dear Out,
The answer is, sort of. The topic "Choosing Folders with the Windows Forms FolderBrowserDialog Component" describes how to grab the folder that is selected in the FolderDialogBrowser SelectedPath property. You would then walk through the files and subfolders for the SelectedPath and add them to the TreeView using the Nodes.Add method.
To copy a node from one TreeView to another, you can use the Clone method of the TreeNode class. The code would look something like this:
TreeView2.Nodes.Add(TreeView1.SelectedNode.Clone)
You can use the Clone method to create an entire forest of TreeViews if you like.
-- Doc Detective
Dear Doc Detective,
I have tried the Clone method as you suggested but the problem is that it adds the subdirectories and files only if the source directory is selected in the expanded mode. If I try to clone when the selected directory is collapsed, then it adds the main directory, but not all the subfolders and files within it and its subfolders. Can you suggest any code to solve this?
-- Still Out on a Limb in Lincoln
Dear Out,
According to the docs for the Clone method, it should also add any sub items; it doesn't make any distinction between expanded or collapsed nodes. The docs are clearly not very clear on this point.
After some experimentation, the Doc discovered that you can get the second Treeview to display the expanded directories and files by calling ExpandAll on the new node before calling Clone:
Dim newNode As New TreeNode()
newNode = TreeView1.SelectedNode
newNode.ExpandAll()
TreeView2.Nodes.Add(newNode.Clone)
It's funny that the docs don't mention this at all?the Doc will make a request to get a better example in the docs.
- Doc D
Doc's Doc Tip(s) of the Day
While the documentation team at Microsoft tries to cover programming topics as comprehensively as possible, there are always a few things that slip through the cracks.
If you can't find what you need in the MSDN Library, you should also search the Microsoft Knowledge Base. New articles are being added all the time, often based on requests for things that are missing from the docs or are incorrect.
When searching for an article in the MSDN Library, you can choose to search both the Library and the Knowledge Base by choosing "MSDN and KB" from the search dropdown.
If you know the number of the Knowledge Base article that you want, you can go directly to it by entering the following URL in your browser, substituting the article number for "308063": http://support.microsoft.com/default.aspx?scid=kb;en-us;308063
Found a topic in Help that doesn't help? Tell the Visual Studio documentation team about it at vsdocs@microsoft.com.
URLs
http://support.microsoft.com/default.aspx?scid=kb;en-us;308063